### Further steps toward Pro TS - Compiling TS to JS - Refining dev workflows - Testing types & validating data - Fullstack type safety - Exploring the TS ecosystem -- ### Goals - Better understand the TS compiler & its output - Configure TS for backend use cases - Use types for testing & data validation - Dip a toe into the deep ocean of open-source TS -- ### Course Project (continued) We're going full-stack! - `event-me` > Vite frontend - `event-me-api`
> Express/Node backend -- ### Ready? # Let's go!
## Compiling TS
-- ```sh cd event-me-api npm i npm run build ``` ```json "scripts" : { // ... "build": "tsc & vite build" } ``` -- ### Squiggle alert! Let's tackle these backend type errors so the code compiles! `src/routes/events.ts` -- ### Exporting/Importing types ```ts // db.ts export type User = //... ``` ```ts // routes/events.ts import type {User} from '../db'; const u: User = //... ``` -- ### Exploring the compiler output Let's a peek at the `dist/` dir that `tsc` generated Try messing with the `target` value... Notice any differences in the output? -- ### Compilers & Configs & Choices, oh my! How should I configure the tsconfig for my project? [TS Handbook >> Choosing Compiler Options](https://www.typescriptlang.org/docs/handbook/modules/guides/choosing-compiler-options.html) -- ### `tsconfig` Bases [github.com/tsconfig/bases](https://github.com/tsconfig/bases) Starter configs for various use cases "DefinitelyTyped for TSConfigs" -- ### Using & extending bases ```sh npm i -D @tsconfig/recommended ``` ```json // ./tsconfig.json { "extends": "@tsconfig/recommended/tsconfig.json", "compilerOptions": { // .... } } ```
### Types & Data Validation 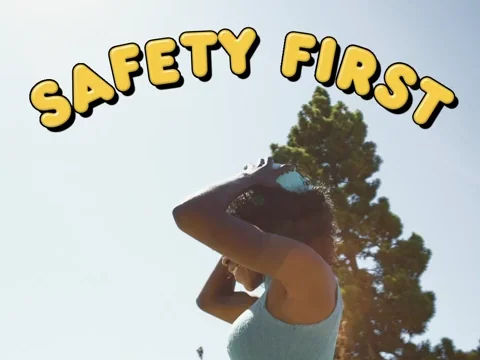 -- ### "end-to-end type safety" 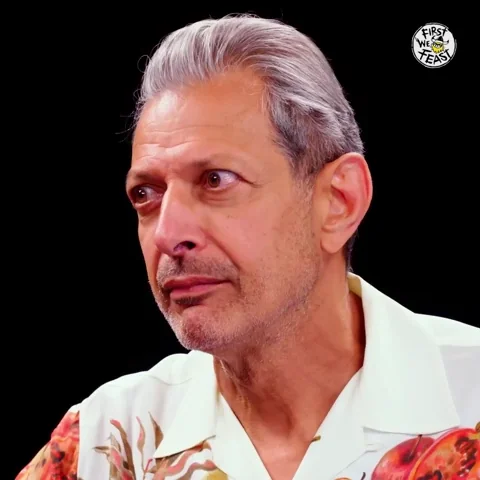 --
-- ### Full-stack typing [TS Handbook >> Project References](https://www.typescriptlang.org/docs/handbook/project-references.html) - Export data types from `event-me-api` modules - Configure TS to link `event-me-api` & `event-me` - Import data types into `event-me` modules -- ### [zod.dev](https://zod.dev) ```sh npm i zod ``` _"TS-first schema validation with static type inference"_ - Provides typed objects to define data schemas - Checks that data has that shape, _at runtime_ in JS - Lets TS infer the matching type(s) -- ### Declaring a Schema ```ts import { z } from "zod"; const User = z.object({ username: z.string(), }); ``` -- ### Validating data ```ts // throws an error if the data // doesn't match the schema User.parse({ username: "Ludwig" }); ``` ### Inferring TS type(s) ```ts // extract the inferred type type User = z.infer
; // { username: string } ``` -- ## Type-aware Tests [vitest.dev/guide/testing-types](https://vitest.dev/guide/testing-types) ```sh npm i -D vitest ``` ```ts import { expectTypeOf } from 'vitest'; import myFn from './myFunction'; import MyType from './sharedSchema'; test('my types work properly', () => { expectTypeOf(myFn).toBeFunction(); expectTypeOf(myFn).not.toBeAny(); expectTypeOf(myFn).parameter(0).toMatchTypeOf
(); }); ``` -- ### Tons of helpful TS libs out there! These are just some popular examples Check out [github.com/dzharii/awesome-typescript](https://github.com/dzharii/awesome-typescript)