### Functionality & Features 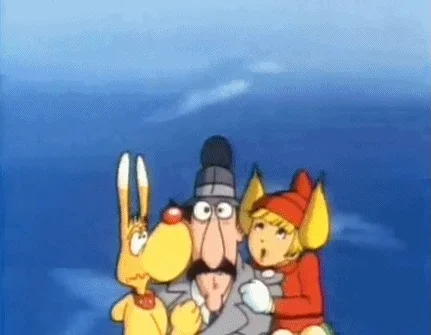 -- ### Goals - Start getting familar with TS syntax - Add types to variables, function arguments & return values - Define & work with with increasingly complex types -- ### Ready? # Let's go!
### Basic Types -- ### Primitive types ```ts string number boolean undefined null ``` -- ### Literal types ```ts const isOpenClass = "modal-is-open"; ``` = -- ### DOM types ```ts HTMLElement HTMLDialogElement MouseEvent FormData ``` -- ### `any` 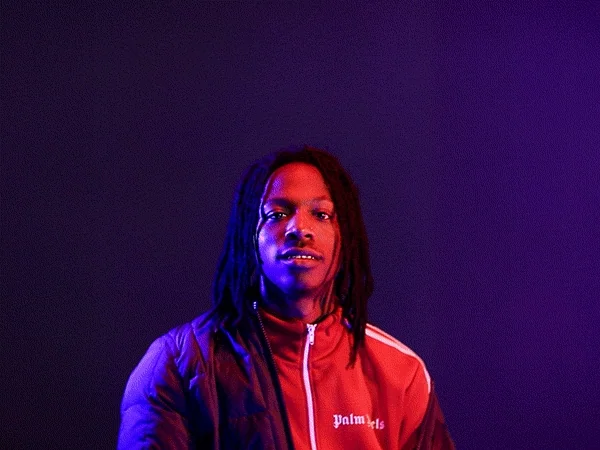 -- ```js // @ts-ignore function (anything) { //... } ``` -- 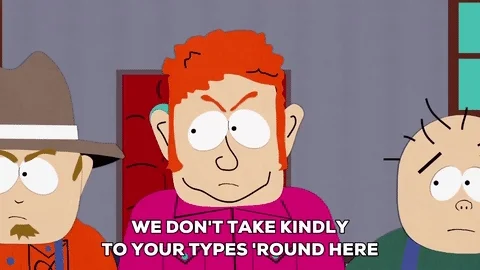
### Type Annotations -- Variables ```ts let x: number = 4; ``` -- Functions ``` function sum(a: number, b: number): number { return a + b; } function concat(a: string, b: string): string { return a + b; } ``` -- ### `void` ```js function nothingReturned(): void { console.log('just logging'); } ``` -- ### `never` ```ts function giveYouUp(): never { throw new Error('Rickrolled'); console.log('never gonna log this down'); } ``` -- ### Union types ```ts let x: number | string; x = 4; // OK x = '4'; // OK let y: number | null; y = 4; // OK y = '4'; // Type 'string' is not assignable to type 'number' y = null; // OK ``` -- ### Typed Arrays ```ts const digits: number[] = [1,2,3]; digits.push(4); // OK digits.push('no go'); // Argument of type 'string' is not // assignable to parameter of type 'number' ``` -- ### Type narrowing & Type guards ```ts const elemOrNull = document.getElementById(myId) if (typeof elemOrNull === 'null') { console.log("null"); return; } else { elemOrNull.addEventListener('click', () => { console.log("HTMLElement"); }) } ``` -- ### Let's get annotatin'! `src/Modal.ts` `src/Forms.ts`
## DIY: Custom types 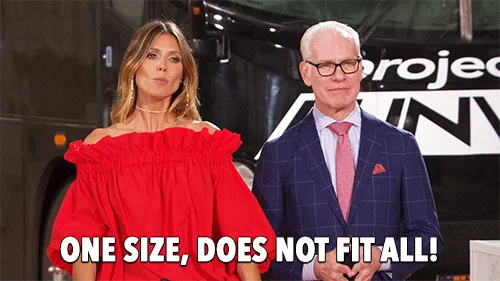 -- ### Type aliases ```ts type StringOrNumber = string | number; type LogLevel = 'log' | 'error' | 'warn'; type MaybeSo = "maybe" | true; type MaybeNot = MaybeSo | undefined | null; ``` -- ### Interfaces ```ts interface User { username: string; email: string; } ``` ```ts interface HumanUser extends User { birthday: Date; } interface AIUser extends User { modelName: string; } ``` -- ### Optional properties ```ts interface Event { name: string; date: Date; host?: User; // User | undefined } ``` -- ### Indexing types ```ts type UserName = User["username"]; // not User[username] or User.username ``` -- ### Generic types ```ts type LogLevel = 'log' | 'error' | 'warn'; function levelLogger
(level: L) { const logger = console[level]; return logger; }; const warn = levelLogger('warn'); warn('Generics can get tricky'); ``` -- ### Let's craft some custom types! `src/Events.ts` - `User` - `Event` - `RSVP`
## We're off and typing! 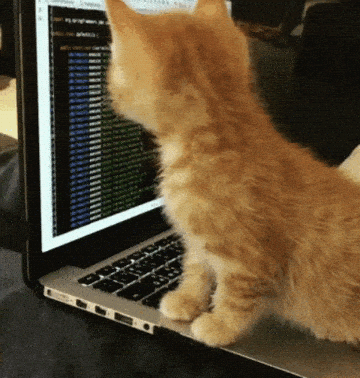 -- We've covered a lot so far: - TS vs JS - Installing & configuring TS - Finding bugs before even adding types - Using primitive & built-in/library types - Creating custom types & interfaces - Unions, Generics & more -- 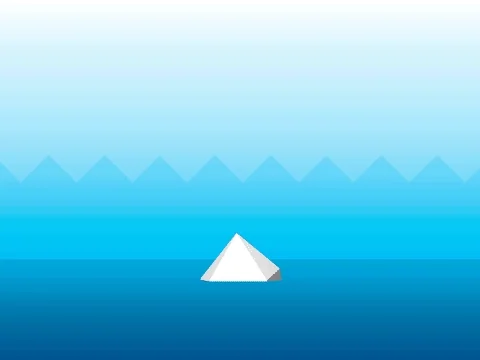 -- ### Coming up: - Compiling TS to JS - Refining your dev workflow - Fullstack type safety - Exploring the TS ecosystem