## TS: Why, What & How
-- ### Goals - Understand the problems TypeScript solves - Install & configure TS in a JS codebase - Start using & compiling TS files -- ### Ready? # Let's go!
## The Errors of our JS Ways -- ### Ever run into errors like this? ```js "Cannot read 'property' of undefined" "Cannot set properties of null" "'undefined' is not a function" ``` -- ### Or things that shouldn't work, but do? ```js document.titel = "My Web Page" // "My Web Page" document.body = 'oopsie'; // undefined document.body // null ``` -- ### Or unexpected results?
-- ### Or just complete nonsense?
"Wat" talk by Gary Bernhardt @ CodeMash 2012
-- What if we could catch problems like this
before
our users do? What if we could even
avoid writing
code that won't run in the first place?
A new
Type
of Script
--
typescriptlang.org/docs
typescriptlang.org/play
### Course Project:
event me!
[github.com/vakila/typescript-first-steps/event-me](https://github.com/vakila/typescript-first-steps/event-me)
-- This JS codebase has some bugs! Let's try to find & fix them with TS! -- ### Setting up - Install VSCode IDE: [code.visualstudio.com](https://code.visualstudio.com/) - Clone/download the project repo:
[github.com/vakila/typescript-first-steps/event-me](https://github.com/vakila/typescript-first-steps/event-me) - Open the project in VSCode ```sh git clone git@github.com:vakila/typescript-first-steps/event-me.git cd event-me code . ``` -- ### Install dependencies ```bash npm install ``` ### Run the dev server ```bash npm run dev ``` ### Open [localhost:5173](http://localhost:5173)
### Time to TypeScript! ```sh npm install --save-dev typescript npm i -D typescript ``` ```sh npx tsc --init ``` --
`tsconfig.json`
-- 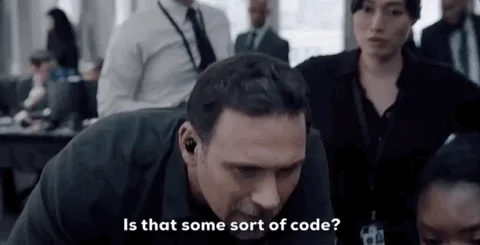 -- ```json { "compilerOptions": { /* Visit https://aka.ms/tsconfig to read more about this file */ } } ``` -- ```json /* Modules */ "module": "ESNext", "rootDir": "./", "moduleResolution": "bundler", "baseUrl": "./", "paths": { "@*": [ "src/*" ], }, ``` -- ```json /* JavaScript Support */ "allowJs": true, "checkJs": true, /* Output options */ "noEmit": true, ``` -- ```sh npx tsc ``` ```json "scripts": [ "check": "tsc" ] ``` -- ![Simpsons gif "I won't be needing this [green pen, only red]"](https://media3.giphy.com/media/v1.Y2lkPTc5MGI3NjExZDU5ajh5bGF4YXZ0YmRqZXF0amFhNzlueHF6ZWg4Mmw5dm0yeHl4eCZlcD12MV9pbnRlcm5hbF9naWZfYnlfaWQmY3Q9Zw/3o6Mb65G4Xjv5j0VGw/giphy.webp) -- ### We need moar types! [Vite](https://vitejs.dev) app uses [Node](https://nodejs.org) to build the site ```json "types": [ "vite/client" ], ``` ```sh npm i -D @types/node ``` -- Now let's go bug hunting! 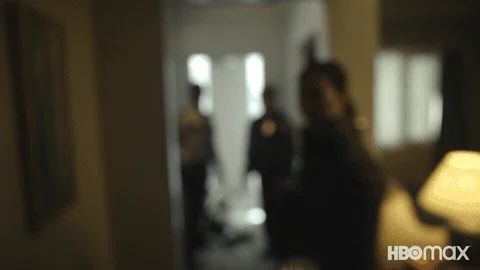
## Better [JS]() with [TS]() [docs/handbook/intro-to-js-ts](https://www.typescriptlang.org/docs/handbook/intro-to-js-ts.html) -- ### Hover away! 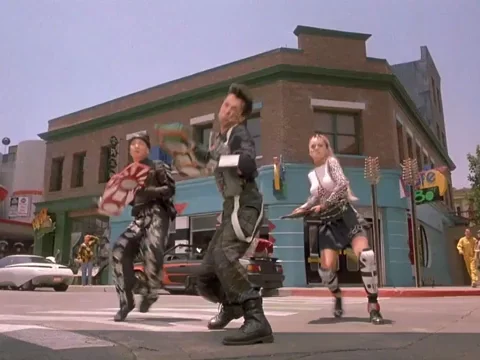 -- ## `src/Header.js` -- ## `src/Modal.js` -- ### From JS to TS ~~`Modal.js`~~
`Modal.ts` -- ### Ready? ## [Let's dive in!](./2.html)